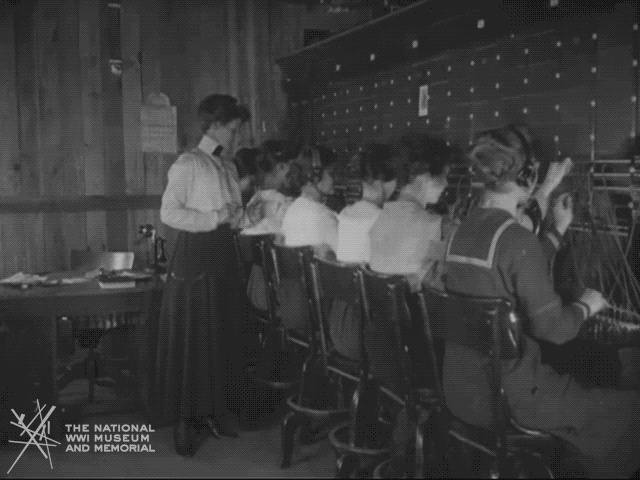
Games have so many moving parts from 3d, animation events, and physics. Not to mention all the code we need to communicate between the different parts and objects.
At this level of complexity, we require ways to handle Dynamic Events between two objects; events such as a sword swing connecting with an enemy, or a player walking close enough for a button prompt to appear. We can not hard-code these setups either as we simply don’t “know” when they will occur.
That’s where GetComponent can come in.

GetComponent
Get component provides us a way to grab any script, or “component” from a gameObject within Unity. This allows us to do things such as grabbing the Rigidbody to dynamically alter gravity off button pushes.
Or for example: telling a specific enemy we struck to tell it to TakeDamage().

GetComponent<T>() is great when we have a reference to any gameObject, and it’s fairly fast executing in the code.
But sometimes we don’t have the exact reference to the object and we have to go hunting for it. Things like game managers we never have the player directly “touch” in-game but rather are pre-configured for our code to talk to.
For this, we need Unity’s Find() command.
GameObject.Find(“Name”)
Now quick disclaimer, this is a COSTLY function. It’s hunting through the entire loaded scene in the hopes of finding a single object. If using on every object with thousands of objects in the scene, it will create an issue.
If we’re simply doing Find() on startup and caching the reference though, it can be a great tool.

We use Find() to first find a gameObject with the name “Game_Manager” and then we use GetComponent<> to get the actual script tied to the gameObject. Then we store the GameManager reference into _gm to later use it.