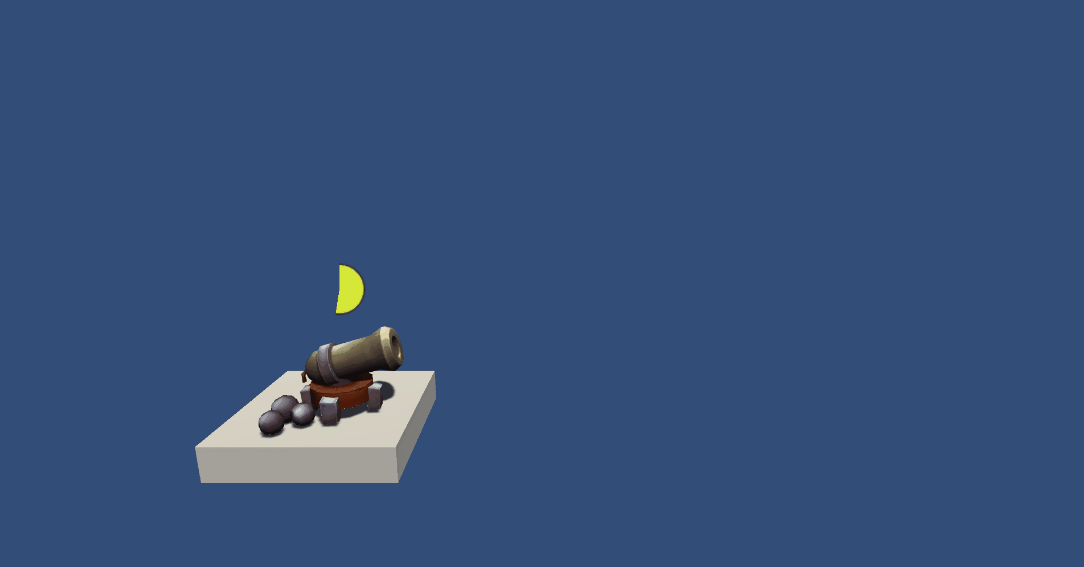
As discussed before timers are everywhere in games. BUT in past articles, I’ve only written about simple timers using delta time in the default Update Loop.
If we look at Unity’s Order of Execution we can see there is something that happens immediately after the update loop called Coroutines. These are extremely powerful once you know what you're doing with them and they work more intuitively to how we think about timing.

What is a Coroutine
Put in the simplest of terms, they work like the update loop but you can tell it to “Wait” a set time before running again. Coroutines can also be dynamically Started, Paused, and Stopped by you in code.
This lets us do several powerful things but they all revolve around “Waiting”.
Wait for x seconds in time to pass, wait for a download to finish, wait on every frame until x happens. Lots of ways to use them.
To demo this, we are going to use a Coroutine to create a simple infinite spawner.
Example Coroutine - Infinite Spawning
This is the “core” Coroutine code we need to have an infinite spawner that works off a given spawn rate (3 seconds in this example)

The steps for a Coroutine are thus:
- We create an IEnumerator return type function
- We put a yield return for the type we want
- Optionally: We put a while(true) in to make the coroutine work forever
- And then we call StartCoroutine(<FunctionName>)
Now the Coroutine will run without a while(true) it will just run once. Additionally, we do not need to call StartCoroutine in Start we can call it anywhere, for example maybe on “PlayerHit” we set a “StayInvincibleFor(3f)” for retro game style invincibility.
From here we just need to do what was covered in my Creating Objects article
- Store prefab we want to spawn
- Give it a spawn position
- Optionally container the spawned object as written about here
Full Example Code
