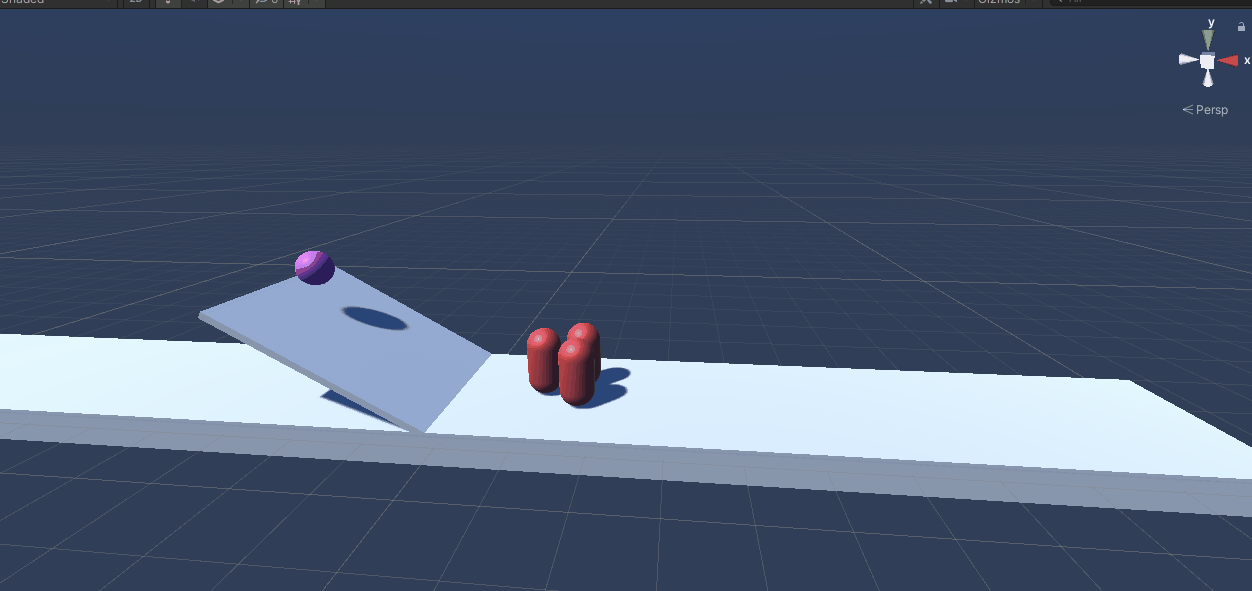
Colliders
The main reason to use any Physics System is you want to know when two objects hit without doing all the math yourself.
The typical ones to use are boxes, capsules, spheres. Sometimes Terrian, and sometimes Mesh. Mesh can become a performance issue if used willy nilly on large models though, so like discussed in part 1, “Close Enough” is good enough. So you might just put a box or capsule around something instead of a Mesh.

3D vs 2D Colliders
Both are great and optimized for their individual roles.
BUT you need to understand the one rule! You can not mix 3D Physics colliders with 2D Physics colliders. This is because they are technically different physics engines which means you need a Rigidbody2D to interact with 2D colliders.
What you are allowed to do, however, is have a 2D Sprite (2D visuals) with a 3D Box Collider (3D physics) interacting with a 3D Rigidbody. Setting up this way would allow you to interact with 3D models, even if the visuals look 2d.
OnCollider vs OnTrigger and How to Use
Colliders engage directly with the physics systems, triggers ignore physics applied to it. Triggers are very useful for “Zones” where you want something to trigger. Maybe an“Activation Zone” before a button prompt pops up and allowing player input.
For either of these events to work, you require at least one of the objects involved to have a Rigidbody. Without this, the physics system doesn’t know it should care about the collider involved.
Example OnTrigger()
To create the trigger we simply need to check the “Is Trigger” box on the Collider and then adjust the collider to fill in the zone we want to fill.
To adjust the collider we can click the “Edit Collider” button and then drag the anchor points, or manually type in the center/size of the collider.

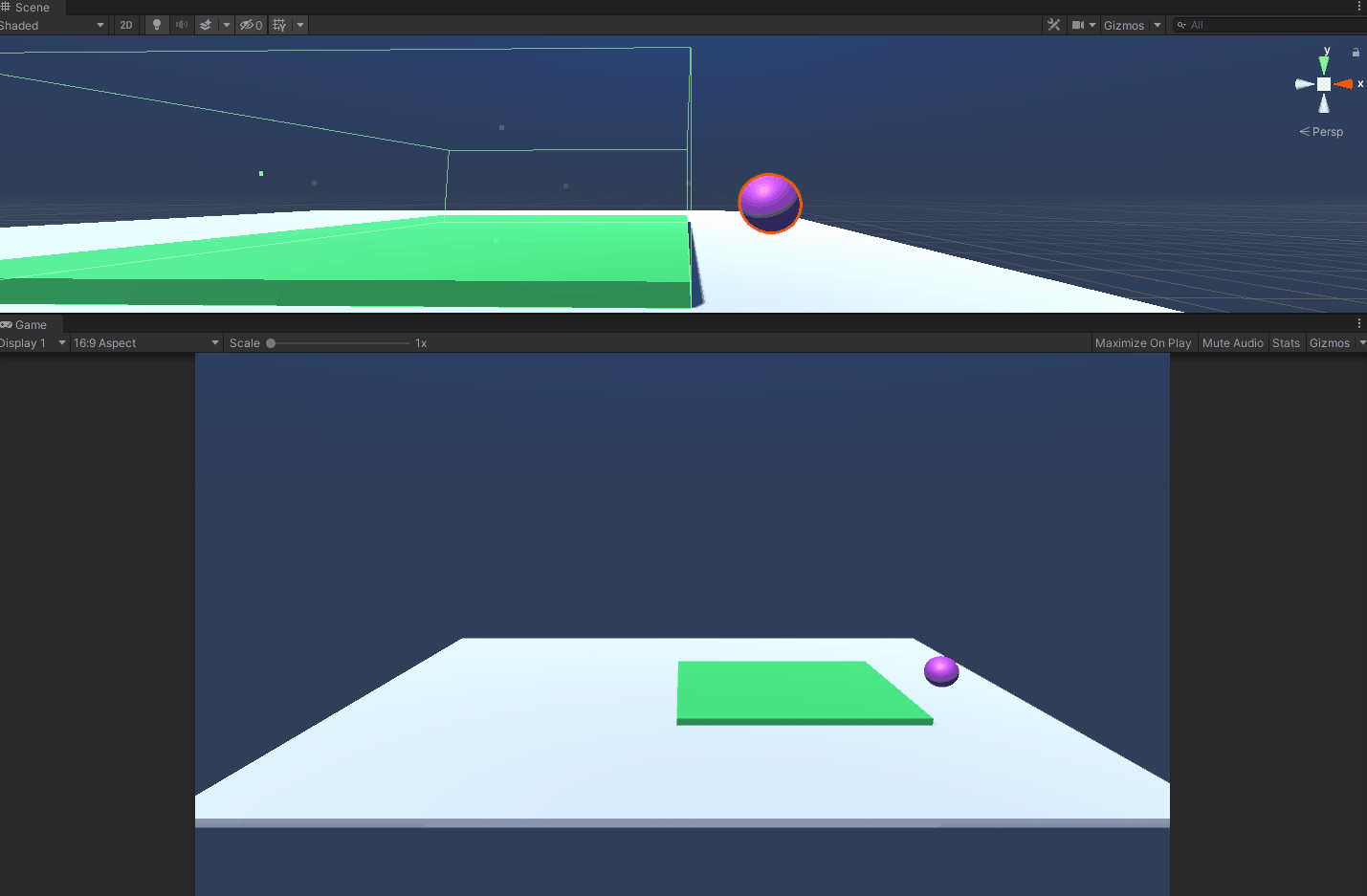
The Trigger Code for this example:

Collisions
Collisions work the same way as triggers but they’ll push back with their own physics settings. The only other difference is in code we use OnCollission.
Now an important thing to learn with OnCollision is making sure you know how to filter out collisions. You don’t want your own projectile attack that’s firing usually close enough to you for example to trigger your collider and damage you for example.
So to avoid hitting our selves with our own attacks, we use Tags or Layers.

This way when the Player shoots a basic projectile if the shot happens to overlap with the player collider on spawn he won’t take damage.

Next Up:
Part 3 will be going over Physics Materials and Part 4 Joints.